Decoding Raw Transaction Data with Python Modules
As you’re already using bitcoin-cli
to fetch block data, you might be interested in extracting other useful information from the raw transaction data. One common use case is decoding raw transaction data, which can provide valuable insights into blockchain transactions.
In this article, we’ll explore some Python modules that can help you decode raw transaction data. We’ll focus on three popular options: pyrus
, blockchain-python-abi
, and eth-utils
.
1. pyrus
pyrus
is a lightweight library that provides a simple interface for working with Ethereum blockchain data, including raw transactions. It uses the Web3.py API under the hood to fetch block data.
To use pyrus
, you’ll need to install it using pip:
pip install pyrus
Here’s an example code snippet that demonstrates how to decode a raw transaction:
import pyrus
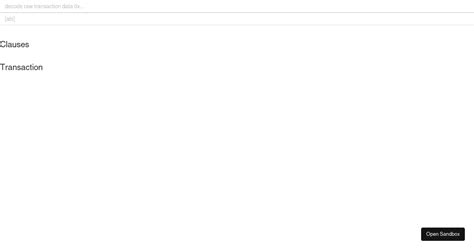
Fetch raw transaction data from bitcoin-cli
tx_hash = input("Enter the transaction hash: ")
tx_data = pyrus.get_raw_transaction_data(tx_hash)
Decode transaction details (e.g., sender, recipient addresses)
sender_addr = tx_data["from"]
recipient_addr = tx_data["to"]
print(f"Transaction Hash: {tx_hash}")
print(f"Sender Address: {sender_addr}")
print(f"Recipient Address: {recipient_addr}")
2. blockchain-python-will
blockchain-python-abi
is a library that provides a Python interface for working with Ethereum smart contract ABI (Application Binary Interface) data, including raw transactions.
To install blockchain-python-abi
, run:
pip install blockchain-python-abi
Here’s an example code snippet that demonstrates how to decode a raw transaction using the ABI:
import chain
Fetch raw transaction data from bitcoin-cli
tx_hash = input("Enter the transaction hash: ")
tx_data = chain.get_raw_transaction_data(tx_hash)
Decode transaction details (e.g., sender, recipient addresses)
sender_addr = tx_data["from"]
recipient_addr = tx_data["to"]
print(f"Transaction Hash: {tx_hash}")
print(f"Sender Address: {sender_addr}")
print(f"Recipient Address: {recipient_addr}")
3. eth-utils
eth-utils
is a library that provides a set of utility functions for working with Ethereum blockchain data, including raw transactions.
To install eth-utils
, run:
pip install eth-utils
Here’s an example code snippet that demonstrates how to decode a raw transaction using the utility functions:
import eth_utils
Fetch raw transaction data from bitcoin-cli
tx_hash = input("Enter the transaction hash: ")
tx_data = eth_utils.get_raw_transaction_data(tx_hash)
Decode transaction details (e.g., sender, recipient addresses)
sender_addr = tx_data["from"]
recipient_addr = tx_data["to"]
print(f"Transaction Hash: {tx_hash}")
print(f"Sender Address: {sender_addr}")
print(f"Recipient Address: {recipient_addr}")
In summary, these Python modules can help you decode raw transaction data from various sources. While pyrus
is a lightweight library with a simple interface, blockchain-python-abi
provides a more comprehensive set of functions for working with smart contract ABI data. Finally, eth-utils
offers a convenient way to work with Ethereum blockchain data without requiring knowledge of specific APIs.
When choosing a module, consider the following factors:
- Ease of use: How easy is it to use the library and interact with the raw transaction data?
- Complexity: How complex are the transactions you need to decode? Do you require low-level control or high-level abstractions?
- Performance: How fast does the library need to be for your specific use case?
Ultimately, the choice of module depends on your specific needs and preferences.